CS110 Lab 19:
Exception Handling and File Input/Output - The Basics
- An exception is an unexpected error condition that prevents the program from continuing normally.
For Example:
- Reading from a file that does not exist.
- Reading from a file that has corrupt data.
- Writing to a file, but the disk/drive is full.
- Reading input from the user and the user enters an invalid data type.
- Unexpected division by zero.
- Accessing an array with a subscript (index) that is out of bounds.
- Applications must be flexible when handling problems, so as not to terminate abruptly.
Exception handling provides an adaptable mechanism for handling such problems.
- Java includes the following common exceptions:
- IOException
- RuntimeException
- ArithmeticException
- IndexOutOfBoundsException
- ArrayIndexOutOfBoundException
- NoSuchElementException
- InputMismatchException
- File IO exceptions are usually "Checked", meaning they are checked at
compile time, such as FileNotFoundException.
Code that uses a checked exception will not compile if the catch is not handled.
- The following is a practical example of exception handling:
Write an app that does the following:
- Writes a sentence to a file. The example below shows a value that is hardcoded,
however, it can be input via the keyboard.
- Read from the file and count the number of words in the sentence.
Display the count to the console.
MyApp.java - Complete the remaining tasks.
import java.io.File;
import java.io.FileNotFoundException;
import java.io.IOException;
import java.io.PrintWriter;
import java.util.Scanner;
public class MyApp {
public static void main(String[] args) {
// _____________________________________________________________
// TASK 1: WRITE A PARAGRAPH TO A FILE
// _____________________________________________________________
// Define a file to be written to, data.txt
File file = new File("data.txt");
// try:
// (a) Create a PrintWriter object to print to a text-output stream.
// (b) Use the PrintWriterWriter object to write a paragraph to the file.
// (c) Close the PrintWriter object when done.
// catch:
// (a) Catch an input/output exception.
// (b) Display an error message for this exception
try {
PrintWriter printWriter = new PrintWriter(file);
printWriter.println("These french fries are cold and delicious!");
printWriter.close();
}
catch (IOException e) {
System.out.println("This file could not be created");
}
// _____________________________________________________________
// TASK 2: READ A PARAGRAPH FROM A FILE
// _____________________________________________________________
// Define a Scanner object.
Scanner fileScan = null;
// try:
// (a) Instantiate the Scanner to read the contents of a file.
// (b) Calls a method to count the words in the file.
// catch:
// (a) Catch an exception if the specified file is not found.
// (b) Display an error message .
// finally:
// (a)Close the Scanner object, if it was properly instantiated.
try {
fileScan = new Scanner(new File("data.txt"));
System.out.print("There are " + count(fileScan) + " words.");
}
catch (FileNotFoundException e) {
System.out.println("Error - This file could not be found.");
}
finally {
if (fileScan != null)
fileScan.close();
}
}
//Method: Reads and counts the words in a file.
public static int count(Scanner fileScan) {
int countItems = 0;
while (fileScan.hasNext()) {
fileScan.next();
countItems++;
}
return countItems;
}
}
Code an app that outputs the largest value from each row in
any 2-dimensional array to a text file and to the console window.
Verify the results.
Note: You may use the hardcoded array below:
int [][] myArray =
{{1,22,5,7,9},
{6,8,23,4,3,6},
{7,8,9,9,9,10,24,3,4},
{3,5,4,7,6,25},
{26,3,4,2,3,2,4}
};
Code an app that produces the following ASCII Bull's Eye to a text file.
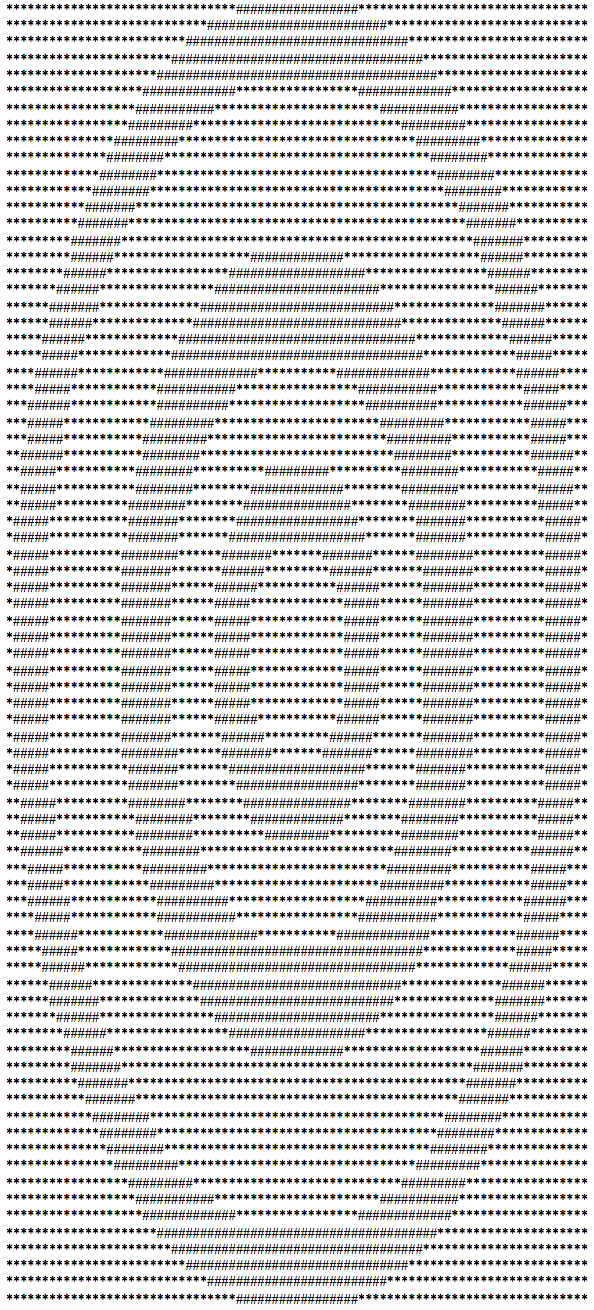