The main objective of this app is to experience Activity callback methods first hand.
Each activity in an Android application goes through its own lifecycle and
relies on the implementation of a series of callback methods that the system
calls when the activity transitions between various states of its lifecycle.
Use your app to perform the experiments shown in the figure below. Produce a Toast pop-up for
each Activity callback method.
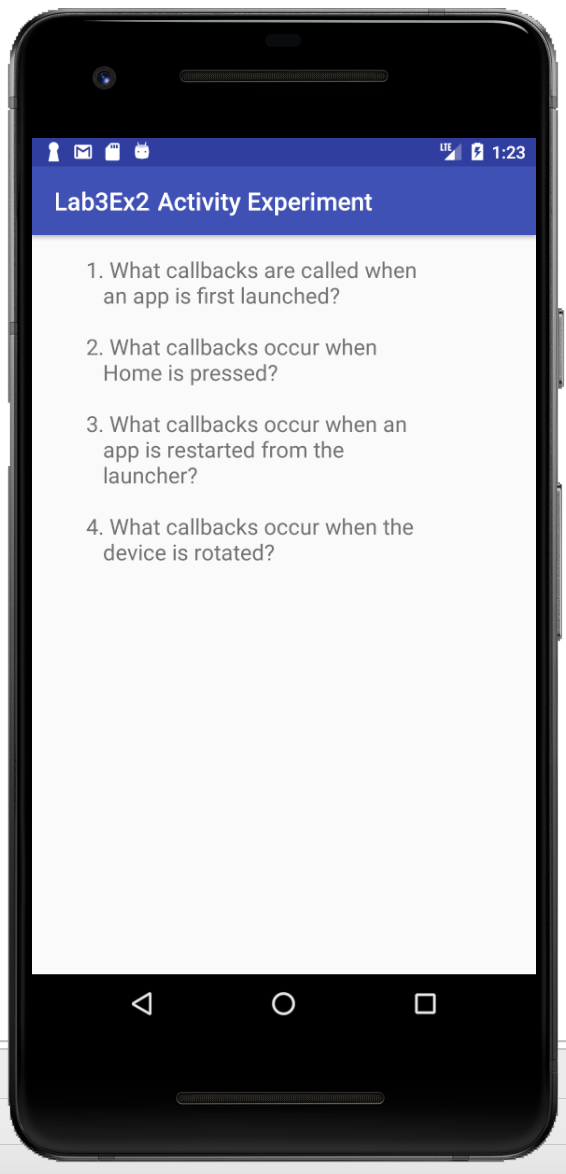
public class MainActivity extends AppCompatActivity {
//CREATE TOAST MESSAGES FOR THE EXPERIMENTS
private String createMsg;
private String startMsg;
private String resumeMsg;
private String pauseMsg;
private String stopMsg;
private String restartMsg;
private String msg;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
initializeToastMsgs();
Toast.makeText(this, createMsg, Toast.LENGTH_LONG).show();
Log.v(msg, createMsg);
}
private void initializeToastMsgs(){
createMsg = "Callback: onCreate()";
startMsg = "Callback: onStart()";
resumeMsg = "Callback: onResume()";
pauseMsg = "Callback: onPause()";
stopMsg = "Callback: onStop()";
restartMsg = "Callback: onRestart()";
msg = "activity callbacks ";
}
//************ override the callbacks to display Toast messages ****************
}
Exercise 2:
Create the app shown below. The goal of this app is to construct an app by researching online
documentation. This app requires MediaPlayer for Android.
Begin by creating a raw directory in the resources directory and loading it with an
interesting sound. Test the app by playing the sound, pausing it, and then continuing to play it.
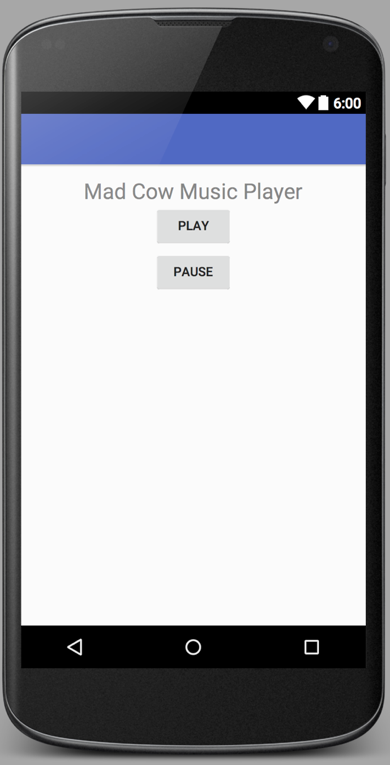
cow_mad_x.wav
Exercise 3:
This exercise explores dynamic additions of Views to a layout.
Create an app that allows the user to build a Fibonacci flower by adding two types
of petals. The app algorithm must position, rotate, and scale each petal
before it is added to the screen. The user should be allowed to erase the
petals to rebuild a new flower. This app is built in the Textbook under lab Example 5-1.
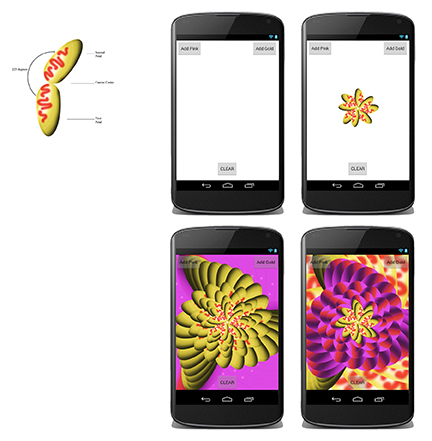
Exercise 4:
Create the simple calculator shown below. This app should use a TableLayout.
NOTE: This app is solved in the textbook under
Lab Example 2-4. Refer to this lab code for guidance.
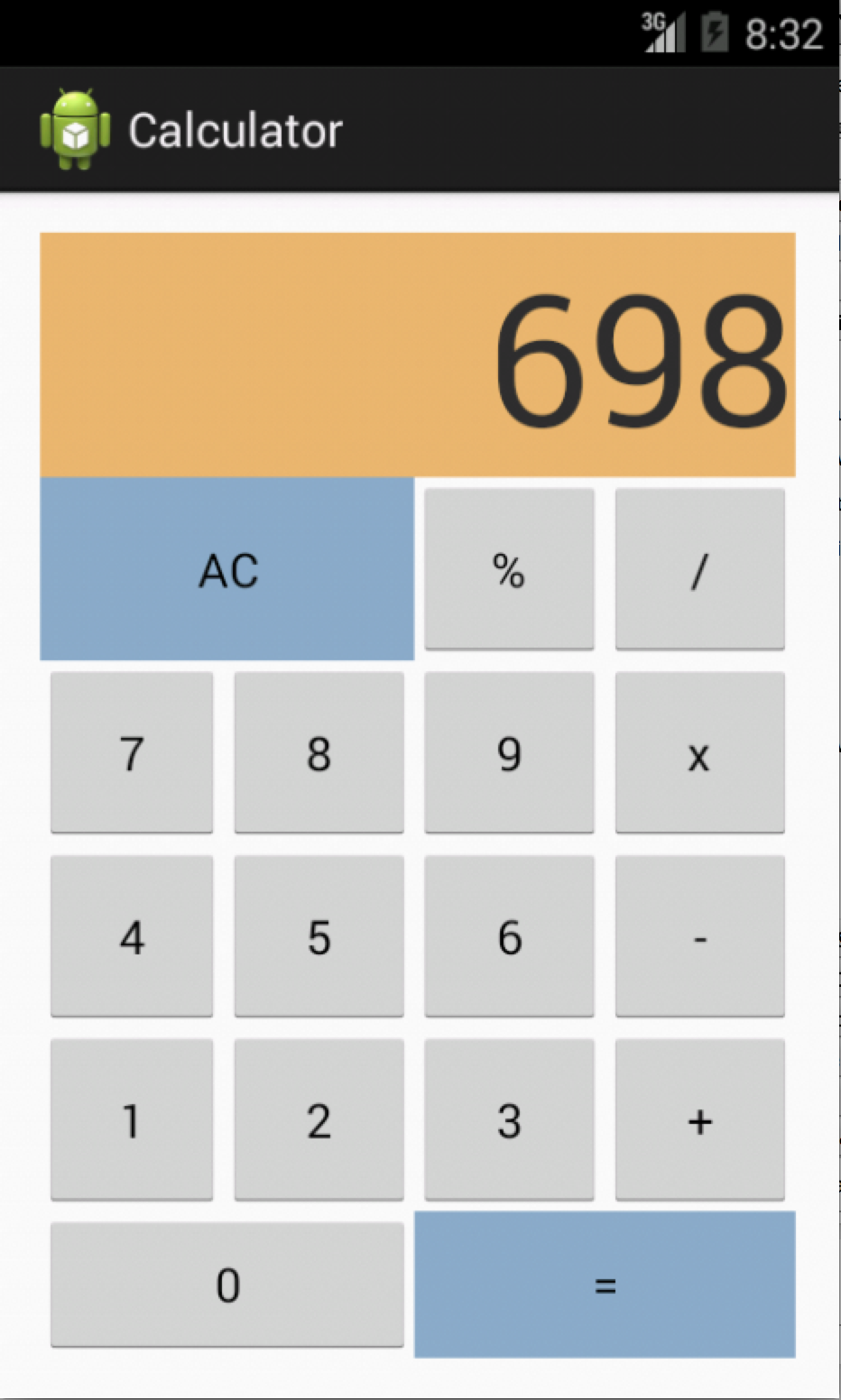
Exercise 5:
The goal of this app is to understand how data can be passed between activities
within the same application.
Consider the Automotive Calculator App in Lab Example 3-3, which utilizes two
user interface screens:
- Input Activity: Allows the user to input car price, down payment and loan period.
-
Loan Summary Activity:This activity is called to display the loan summary.
It is passed the computed loan information and displays information in a scollable format.
Build this app, which is solved in the textbook.
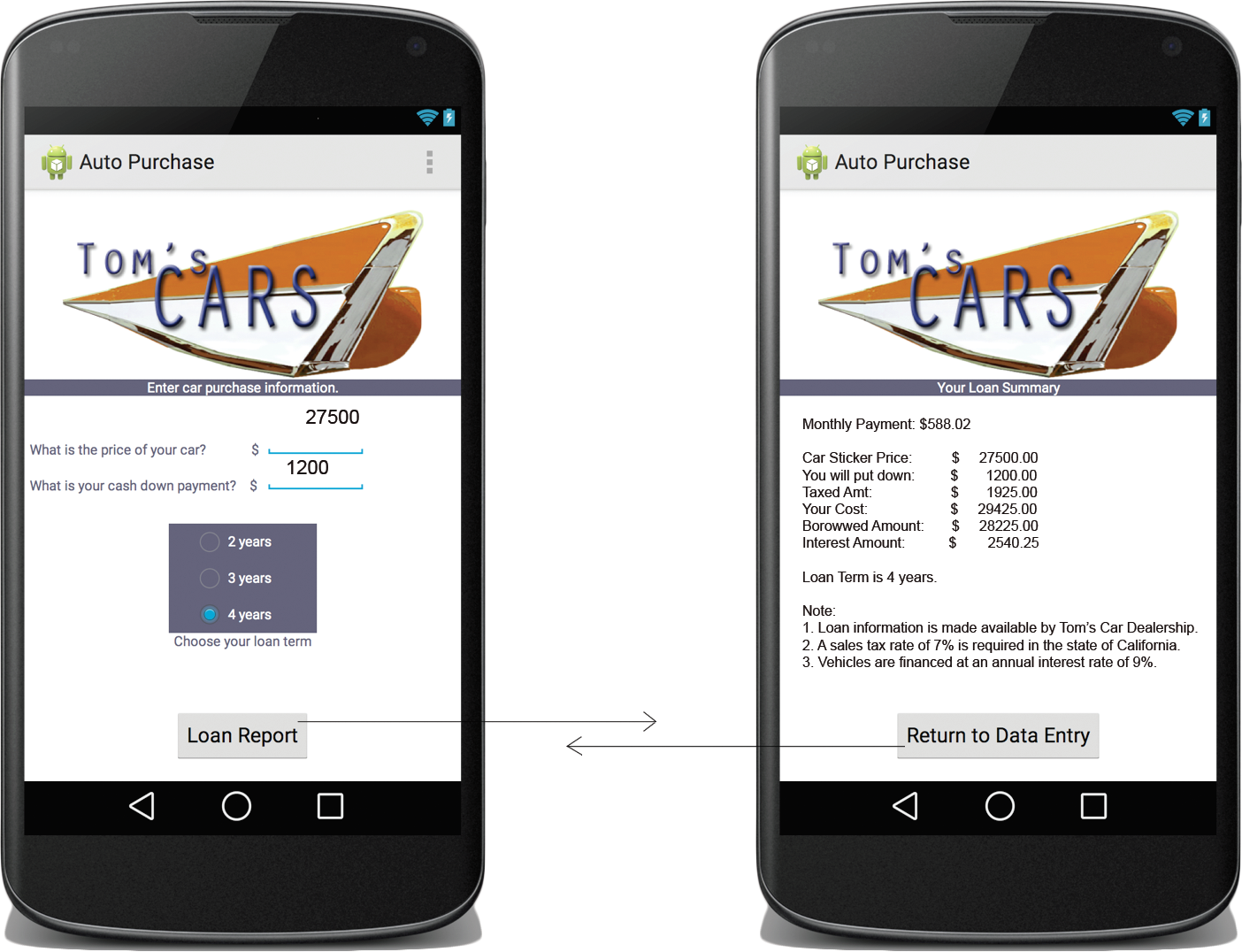